Saturday 30 May, 2009
Getting started with jQuery
Key Features of jQuery
- Support for Browser independence: jQuery is supported by most of the modern day browsers.
- Support for a simplified Event Handling model: jQuery provides support for an excellent, easy to use normalized event handling model with much reduced code. The jQuery Event Handling model is consistent across all browsers. The even object is a cross browser normalized one and one event object is always passed as a parameter to an event handler.
- Support for Seamless extensibility: jQuery provides support for extensibility through an easy to use plug-in API that enables extending the jQuery core library seamlessly.
Pre-requisites
To get started with jQuery you should have the following installed in your system:
- Visual Studio 2008
- Visual Studio 2008 SP1
- jQuery Library
- Visual Studio 2008 jQuery Plug-in
Downloading jQuery
You can download the jQuery library from this link: http://jquery.com/
As of this writing, the current version of jQuery is 1.3.2
Getting started with jQuery
In this section, we will explore how we can get started with jQuery in ASP.NET in a step-by-step manner.
Note: To get a feel of jQuery intellisense in Visual Studio 2008, you should install the HotFix: KB958502 that comes free from Microsoft. You should also download the jQuery Intellisense file and place it side by side along with the jQuery library in your Visual Studio solution, i.e., place it in the same path.
To get started with your first sample application in ASP.NET that uses jQuery, follow these steps:
1. Open Visual Studio and click on File-> New->Project.
2. Select .NET Framework 3.5 as the version and the ASP.NET Web Application template from the list of templates displayed.
3. Specify a name for the project and click OK to save.
4. Create a new folder in the solution explorer and name it as Scripts.
5. Right-click on this folder and select Add Existing Item.
6. Now, browse to the path where you have downloaded the jQuery library and the jQuery intellisense documentation.
7. Select the two files and click on Add to add the files in the Scripts folder.
8. Next, drag and drop the jquery-1.2.6.js file from the Solution Explorer on to the Head section of the Default.aspx file to create a reference as shown below:
1 "server"> 2 Getting Started 3 4
9. Now add the following script to your web page:
1 |
The Complete Example
Here is the complete code for your reference:
1 <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="_Default" %> 2 > 3 <html xmlns="http://www.w3.org/1999/xhtml" > 4 <head runat="server"> 5 <script src="Scripts/jquery-1.2.6.js" type="text/javascript">script> 6 <title>Getting Startedtitle> 7 <script type="text/javascript"> 8 $(document).ready(function() 9 { 10 alert("Using jQuery in ASP.NET"); 11 }); 12 script> 13 head> 14 <body> 15 <form id="form1" runat="server"> 16 form> 17 body> 18 html>
1 | <%@ Page Language="C#" AutoEventWireup="true" CodeBehind="Default.aspx.cs" Inherits="_Default" %> |
2 | > |
3 | <html xmlns="http://www.w3.org/1999/xhtml" > |
4 | <head runat="server"> |
5 | <script src="Scripts/jquery-1.2.6.js" type="text/javascript">script> |
6 | <title>Getting Startedtitle> |
7 | <script type="text/javascript"> |
8 | $(document).ready(function() |
9 | { |
10 | alert("Using jQuery in ASP.NET"); |
11 | }); |
12 | script> |
13 | head> |
14 | <body> |
15 | <form id="form1" runat="server"> |
16 | form> |
17 | body> |
18 | html> |
Summary
jQuery is a simple, light-weight and fast JavaScript library that has simplified the way you write your scripts these days. It is all set to become the framework of choice for building fast and responsive web applications in the years to come.For additional information on jQuery :
Ajax with JQuery - An Introduction with jQuery
Ajax with JQuery - An Introduction with jQuery
jQuery is a well-known and famous lightweight, fast and concise JavaScript Library. jQuery simplifies various task for us like HTML document traversing, event handling, animating, and Ajax interactions for rapid web development. jQuery is great library to reduce our JavaScript coding so they quote "jQuery: The Write Less, Do More".
jQuery was released on January 2006 at BarCamp NYC by John Resig under the MIT License and the GNU General Public License, jQuery is free and open source software. jQuery is very versatile and easy to use, provides great flexibility in designing and development. Considering such great features of jQuery both Microsoft (jQuery will be distributed with Visual Studio) and Nokia (jQuery to develop applications for their WebKit-based Web Run-Time) are taking the major step of adopting jQuery as part of their official application development platform.
You can download the lattest version from jQuery's official homepagehttp://jquery.com/ . There are two version of jQuery available for download freely. For the Production point of view minified version which is in compressed form and Development point of view Uncompressed Code form.
Is jQuery faster than JavaScript?
jQuery is framework and JavaScript is a client side scripting language. jQuery is written in JavaScript and therefore cannot be "faster" than JavaScript. However, jQuery certainly makes your code much more elegant and sophisticated. jQuery provides functionality to deal pretty with CSS, DOM to select a set of desired elements and apply any functionality to it. If you are curious about how jQuery does this and eager to learn it, we are going to see the working of jQuery in future articles.
jQuery or JavaScript?
jQuery is compact and light weight. It also helps to reduce length of your code. We are able to add lots of effect like transition, animation and Simplify common JavaScript tasks, Modify the appearance of a page with Galleries, slideshows, News rotator, sliders and many more. So I like to suggest using jQuery will be better as compare to JavaScript. As jQuery is framework written in JavaScript means in other word you are going to use JavaScript but in the form of jQuery.
Which Framework should I Use?
You are free to use any framework you like. There is a debate in the Web Developer communities; Which framework is better, and to choose for your own projects
Can I use jQuery with other library?
The jQuery library, and virtually all of its plugins are constrained within the jQuery namespace. jQuery uses "$" as a shortcut for "jQuery". Using "$" can override and may cause issues but you can solve it by calling jQuery.noConflict(), so you shouldn't get a clash between jQuery and any other library like Prototype, MooTools, or YUI.
Friday 8 May, 2009
What is the difference between Int.parse and Convert.ToInt32
For example,
string str = "15";
int Val1= Convert.ToInt32(str);
int Val2= int.Parse(str);
From the above code, both Val1 and Val2 return the value as 15. Suppose the string value str has null value, Convert.ToInt32 will return zero and int.Parse will throw ArgumentNullException error.
For example,
string str=null;
int Val1= Convert.ToInt32(str);
int Val2 = int.Parse(str); //This will throw ArgumentNullException Error
Tuesday 5 May, 2009
Run IE6, IE7, and IE8 on the same PC!
I.IETester (For the IE problem specifically) :
IETester is a free WebBrowser that allows you to have the rendering and javascript engines of IE8, IE7 IE 6 and IE5.5 on Vista and XP, as well as the installed IE in the same process.
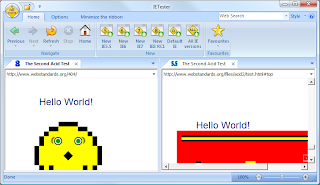
http://www.my-debugbar.com/wiki/IETester/HomePage
II. XenoCode :
Meet Xenocode. Xenocode is basically a way to virtualize applications. This product allows software developers to package applications into a self-contained executable that can be run directly without having to install anything.
Xenocode has decided to demo the technology by creating a browser sandbox. They've created free virtualized versions of Internet Explorer 6, 7, and 8; Mozilla Firefox 2 and 3; Apple Safari; Google Chrome; and Opera.
Finally! You can now easily run multiple versions of Internet Explorer on the same computer!